Manual Devices
Connect to the Arduino Cloud any kind of device that uses Python, MicroPython or JavaScript (Node.js)
Authentication & data synchronisation is automatically handled when you choose the automatic configuration option in the Arduino Cloud.
You can during the setup of your device instead choose the manual configuration option. This allows you to connect to the Cloud using the Device API (MicroPython, Python or Node.js).
This opens up possibilities for more kinds of devices, mainly Linux based, to connect to the Arduino Cloud.
Manual configuration is recommended for more advanced users, mainly those that are looking to integrate existing projects with the Arduino Cloud.
Goals
In this article you will learn how to configure a manual device, and how to connect to the Arduino Cloud with:
- MicroPython
- Python
- JavaScript (Node.js)
Hardware & Software Needed
Depending on what framework you choose to connect with, you will need various requirements.
- To connect using MicroPython you need to have a GIGA R1 WiFi, Portenta H7, Nano RP2040 Connect with MicroPython => 1.2 installed.
- To connect using Python you need to have a version of Python installed on your machine (check
). This has been tested with Python version 3.11.python --version
- To connect using JavaScript, you will need to install Node.js is required to be installed to connect via JS.
Each method of interaction with the Device API requires various levels of complexity.
Device API
The Device API allows you to interact with the Arduino Cloud MQTT broker, by sending/receiving updates from your Arduino Cloud variables.
This API is ideal for you who'd like to integrate existing Python or JavaScript projects with Arduino.
This API is currently split between two repositories:
- arduino-iot-js - for Node.js / JavaScript
- arduino-iot-cloud-py for Python / MicroPython
Note that the Device API is designed to interact with the MQTT broker. To manage devices, Things etc., please refer to the Application API which can be interacted with using HTTP requests.
Configure Manual Devices
To configure a manual device, go to devices in the Arduino Cloud, and click the "Add" button. This will open a new window, where you will be asked to either configure automatically, or manually. Choose the "Manual" option.
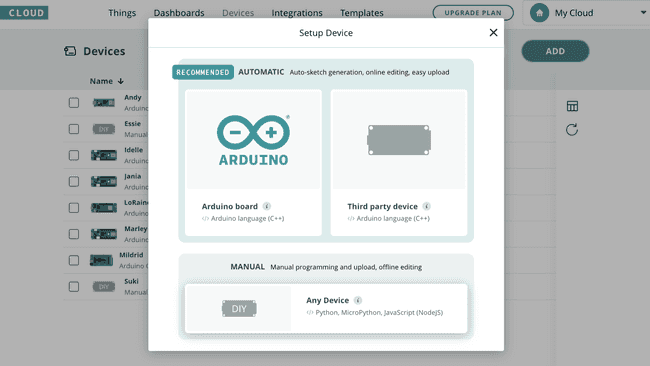
Follow the steps (name your device), and at the end of the wizard you will receive your credentials, which you can also download as a PDF.
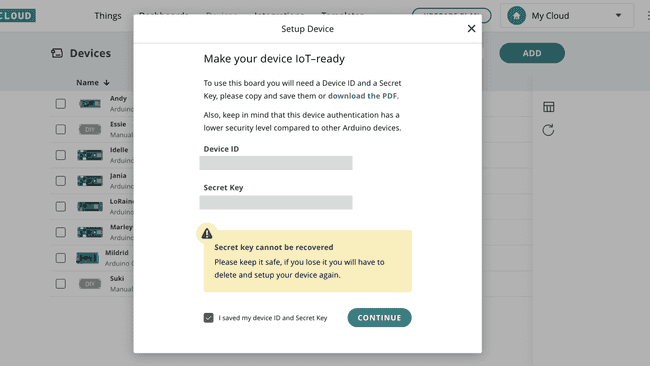
Make sure to save the credentials, as the Secret Key will no longer be obtainable after completing the installation.
After you have created your device, you need to link it to a Thing before using it. This is done in the Thing interface, under "Associated Devices".
MicroPython
The pre-requisities for connecting the the Arduino Cloud via MicroPython are:
- A GIGA R1 WiFi / Portenta H7 board with MicroPython installed,
- Arduino Lab for MicroPython code editor,
- arduino-iot-cloud-py installed,
To install MicroPython, you can check out the Installation Guide. The installation process is the same for both boards as they are based on the same MCU (STM32H7).
To connect with MicroPython, you will need to have a manual device created in the Arduino Cloud. When you create it, you will receive a Device ID and a Secret Key.
MicroPython Example
Below is a script that connects to the Cloud, and allows you to control the onboard LED from a dashboard in the Cloud. You will need to have exactly matching variables created inside your Thing in the Cloud for this to work. For example:
requires a variable namedclient.register("led", value=None)
.led
1from machine import Pin2import time3import network4import logging5from arduino_iot_cloud import ArduinoCloudClient6
7WIFI_SSID = "WIFI_NETWORK"8WIFI_PASSWORD = "WIFI_PASSWORD"9DEVICE_ID = "YOUR_DEVICE_ID"10SECRET_KEY = "YOUR_SECRET_KEY"11
12led = Pin("LEDB", Pin.OUT) # Configure the desired LED pin as an output.13
14def on_switch_changed(client, value):15 # Toggles the hardware LED on or off.16 led.value(not value)17 18 # Sets the value of the Cloud variable "led" to the current state of the LED19 # and thus mirrors the hardware state in the Cloud.20 client["led"] = value21
22def wifi_connect():23 if not WIFI_SSID or not WIFI_PASSWORD:24 raise (Exception("Network is not configured. Set SSID and passwords in secrets.py"))25 wlan = network.WLAN(network.STA_IF)26 wlan.active(True)27 wlan.connect(WIFI_SSID, WIFI_PASSWORD)28 while not wlan.isconnected():29 logging.info("Trying to connect. Note this may take a while...")30 time.sleep_ms(500)31 logging.info(f"WiFi Connected {wlan.ifconfig()}")32
33if __name__ == "__main__":34 # Configure the logger.35 # All message equal or higher to the logger level are printed.36 # To see more debugging messages, set level=logging.DEBUG.37 logging.basicConfig(38 datefmt="%H:%M:%S",39 format="%(asctime)s.%(msecs)03d %(message)s",40 level=logging.INFO,41 )42 43 # NOTE: Add networking code here or in boot.py44 wifi_connect()45 46 # Create a client object to connect to the Arduino Cloud.47 # For MicroPython, the key and cert files must be stored in DER format on the filesystem.48 # Alternatively, a username and password can be used to authenticate:49 client = ArduinoCloudClient(device_id=DEVICE_ID, username=DEVICE_ID, password=SECRET_KEY)50
51 # Register Cloud objects.52 # Note: The following objects must be created first in the dashboard and linked to the device.53 # This Cloud object is initialized with its last known value from the Cloud. When this object is updated54 # from the dashboard, the on_switch_changed function is called with the client object and the new value.55 client.register("ledSwitch", value=None, on_write=on_switch_changed, interval=0.250)56
57 # This Cloud object is updated manually in the switch's on_write_change callback to update the LED state in the Cloud.58 client.register("led", value=None)59
60 # Start the Arduino Cloud client.61 client.start()
For a more details, you can visit a more complete guide at Connecting to Arduino Cloud using MicroPython.
Python
The pre-requisities for connecting with Python is:
- Python installed on your machine (this is tested and confirmed to work with v3.11),
- arduino-iot-cloud-py installed,
- a Thing + manual device created in the Arduino Cloud.
Connection to the Cloud via Python uses the same API as the MicroPython example listed in this article. To install the arduino-iot-cloud-py module, we can use
pip
.1pip install arduino-iot-cloud
You will also need to install SWIG, which is also done via
pip
:1pip install swig
You will also need to have configured a manual device in the Cloud. The Device ID and Secret Key are required in your script to authenticate. To connect, we use the following command:
1client = ArduinoCloudClient(device_id=DEVICE_ID, username=DEVICE_ID, password=SECRET_KEY)
To use the script further below, you will need to create a Thing with the following variables:
- booleanledSwitch
- floattemperature
The variables are set up to test bi-directional communication between the Cloud and the manual device. The
temperature
variable will send just a dummy value to the Cloud, and the ledSwitch
will send data from the Cloud to the manual device.In the script, we need register the variables to use them, and set some parameters.
1# register and send "temperature" data2client.register("temperature") 3client["temperature"] = 204
5# register and set callback (on_switch_changed) 6client.register("ledSwitch", value=None, on_write=on_switch_changed)
Python Example
Below is a script that you can use to test out the API. Make sure to replace the
DEVICE_ID
and SECRET_KEY
entries with your own credentials.1import time2import logging3
4import sys5sys.path.append("lib")6
7from arduino_iot_cloud import ArduinoCloudClient8
9DEVICE_ID = b"YOUR_DEVICE_ID"10SECRET_KEY = b"YOUR_SECRET_KEY"11
12def logging_func():13 logging.basicConfig(14 datefmt="%H:%M:%S",15 format="%(asctime)s.%(msecs)03d %(message)s",16 level=logging.INFO,17 ) 18
19# This function is executed each time the "ledSwitch" variable changes 20def on_switch_changed(client, value):21 print("Switch Pressed! Status is: ", value)22
23if __name__ == "__main__":24
25 logging_func()26 client = ArduinoCloudClient(device_id=DEVICE_ID, username=DEVICE_ID, password=SECRET_KEY)27
28 client.register("temperature") 29 client["temperature"] = 2030 client.register("ledSwitch", value=None, on_write=on_switch_changed)31 32 client.start()
Once you run the script, you will start the client and you will be able to interact with it from a dashboard. The script is setup so that anytime the
ledSwitch
changes, we will print the current state of the variable (true/false) in the REPL. JavaScript/Node.js
The pre-requisities for connecting with Node.js is:
- Node.js installed on your machine (this is tested and confirmed to work with v20.2.0),
- arduino-iot-js installed,
- a Thing created in the Arduino Cloud,
- a manual device created in the Arduino Cloud, associated to your Thing.
Connection to the Cloud via Node.js/Javascript requires you to first install the arduino-iot-js package. You will also need to configure a manual device in the Cloud, which will generate the Device ID and Secret Key needed to connect.
1npm install arduino-iot-js
After installation, you can use the example below to connect and send variable updates to the Cloud.
JavaScript Example
This example connects to the Cloud (MQTT broker), and sends a variable update with
sendProperty()
, and then listens for updates using the onPropertyValue()
method.Please note:
needs to contain the variable name you create in the Arduino Cloud. In this case, we are calling it cloudVar
test_variable
1const { ArduinoIoTCloud } = require('arduino-iot-js');2
3(async () => {4 const client = await ArduinoIoTCloud.connect({5 deviceId: 'YOUR_DEVICE_ID',6 secretKey: 'YOUR_SECRET_KEY',7 onDisconnect: (message) => console.error(message),8 });9
10 const value = 20;11 let cloudVar = "test_variable"12
13 client.sendProperty(cloudVar, value);14 console.log(cloudVar, ":", value);15
16 client.onPropertyValue(cloudVar, (value) => console.log(cloudVar, ":", value));17})();
On successful connection, we should receive the following:
1found association to thing: <thingid>
And upon receiving an update (if you change the value in the dashboard), you should see:
1test_variable: <value>
Summary
In this article, you have learned about the Arduino Cloud's Device API, and how to connect to it using MicroPython, Python & JavaScript (Node.js).
This API makes it easier to integrate existing software projects written in Python & JavaScript.
Suggest changes
The content on docs.arduino.cc is facilitated through a public GitHub repository. If you see anything wrong, you can edit this page here.
License
The Arduino documentation is licensed under the Creative Commons Attribution-Share Alike 4.0 license.